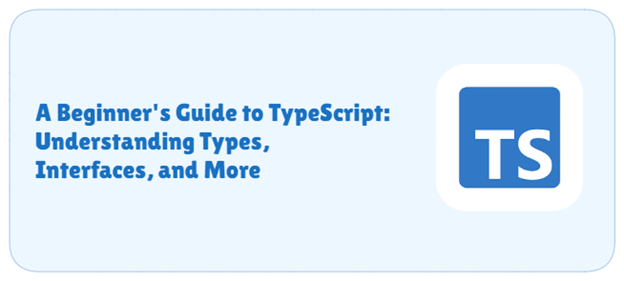
TypeScript is a powerful tool for developers looking to build scalable, maintainable, and error-free applications. Developed by Microsoft, TypeScript is a superset of JavaScript that introduces static typing to the language. This addition provides developers with the ability to catch errors early in the development process, leading to more robust and reliable codebases.
What is TypeScript?
TypeScript extends JavaScript by adding optional static typing, which allows developers to define the types of variables, function parameters, and return values. Unlike JavaScript, which is dynamically typed, TypeScript lets you enforce type constraints at compile time, reducing runtime errors.
Core Features of TypeScript
- Static Typing: Helps catch errors at compile time rather than runtime.
- Modern JavaScript Features: Supports the latest JavaScript features and offers backward compatibility.
- Type Inference: Automatically infers types to simplify code without sacrificing safety.
- Tooling Support: Works seamlessly with IDEs, offering features like autocompletion, refactoring, and error checking.
TypeScript files use the .ts extension and are transpiled into plain JavaScript to ensure compatibility with any environment that runs JavaScript. Its combination of strong typing and developer-friendly features makes it a go-to choice for creating scalable applications.
Here’s an example:
let greet = “Good Morning!”; hello = 55; // Error: Type ‘number’ is not assignable to type ‘string’. let greet_india = “Namaste!”; greet_india = “Suprabhat!”; // No Error |
Steps to Set Up TypeScript in Your Project:
Setting up TypeScript in your project is straightforward. Here’s a step-by-step guide:
1. Install Node.js
Before getting started, ensure you have Node.js installed on your system. TypeScript relies on Node.js for package management using npm or yarn.
2. Initialize Your Project
If you don’t already have a project, create one and initialize it with a package.json file:
mkdir my-typescript-project cd my-typescript-project npm init -y |
3. Install TypeScript
Install TypeScript as a development dependency:
npm install typescript –save-dev |
4. Set Up a TypeScript Configuration File
Generate a tsconfig.json file by running:
npx tsc –init |
This file contains all the configuration options for TypeScript. The generated file includes default settings, but you can customize it based on your needs. For example:
{ “compilerOptions”: { “target”: “ES6”, “module”: “CommonJS”, “strict”: true, “outDir”: “./dist”, “rootDir”: “./src” } } |
- target: Specifies the JavaScript version to compile to (e.g., ES6).
- module: Defines the module system (e.g., CommonJS for Node.js).
- strict: Enables strict type checking.
- outDir: Specifies where compiled files will be placed.
- rootDir: Indicates the source directory for your TypeScript files.
5. Create Your Project Structure
Organize your project files. For example:
my-typescript-project/ ├── src/ │ └── index.ts ├── dist/ ├── package.json ├── tsconfig.json |
Write your TypeScript code in the src directory.
6. Write Your First TypeScript File
Create a file src/index.ts and add some TypeScript code:
const greet = (name: string): string => { return `Hello, ${name}!`; }; console.log(greet(“TypeScript”)); // Hello, TypeScript! |
7. Compile TypeScript to JavaScript
Run the TypeScript compiler to convert your .ts files into .js files:
npx tsc |
The output JavaScript files will be in the dist folder (as specified in tsconfig.json).
To compile everything and watch for changes:
tsc -w |
8. Run the Compiled JavaScript
Use Node.js to execute the compiled JavaScript:
node dist/index.js |
Types in TypeScript
TypeScript introduces static typing, allowing developers to define the type of variables, function parameters, and return values. Here’s an overview of the main types in TypeScript:
1. Primitive Types
These are basic types like numbers, strings, and booleans.
let age: number = 25; let name: string = “Alice”; let isStudent: boolean = true; let roll_no: number; // Declare variable without assigning a value roll_no = 42; |
2. Array Types
An array can hold a collection of items of the same type.
let numbers: number[] = [1, 2, 3, 4]; // can only contain numbers let names: string[] = [“Alice”, “Bob”]; // can only contain strings let objects: object[] = [{ Alice: 1, Bob: 2 }]; numbers[1] = 20; // No Error numbers[2] = “foo”; // Error: Type ‘string’ is not assignable to type ‘number’ objects[1] = { name: “Alice” }; // No Error objects[2] = 42; // Error: Type ‘number’ is not assignable to type ‘object’ |
3. Union Types
Union types allow a variable to hold more than one type.
let id: string | number; id = “abc123”; // valid id = 123; // valid id = true; // Type ‘boolean’ is not assignable to type ‘string | number’. |
4. Any Type
The any type allows a variable to hold any type of value. It should be avoided for type safety.
let value: any = “Hello”; value = 42; // valid let arr: any[] = [1, “string”, false]; // valid |
5. Void Type
Used for functions that don’t return a value.
function logMessage(message: string): void { console.log(message); } |
6. Never Type
Represents values that never occur, typically for functions that throw errors or never return.
function throwError(message: string): never { throw new Error(message); } |
7. Object Type
Defines the structure of an object.
let user: { name: string; age: number; isStudent: boolean; }; user = { name: “John Doe”, age: 20, isStudent: true, }; user.isStudent = “No”; // Error: Type “No” is not assignable to type ‘boolean’ // Error: Property ‘isStudent’ is missing. user = { name: “Jane Doe”, age: 25, }; |
Functions in TypeScript
Functions are one of the fundamental building blocks of any programming language, and TypeScript enhances them with type safety. By specifying the types of inputs (parameters) and outputs (return values), TypeScript ensures that functions behave as expected, reducing runtime errors and improving code maintainability.
1. Defining Functions
In TypeScript, you can define functions with specific types for parameters and return values. Here’s the syntax:
function name(param1: Type, param2: Type): ReturnType { // function body } |
2. Basic Examples:
- Basic Function with Typed Parameters and Return Value
function add(a: number, b: number): number { return a + b; } console.log(add(2, 3)); // Output: 5 |
- Optional Parameters: Parameters can be marked as optional using the ? symbol:
function greet(name?: string): string { return name ? `Hello, ${name}!` : “Hello!”; } console.log(greet()); // Output: Hello! console.log(greet(“Alice”)); // Output: Hello, Alice! |
- Default Parameters: You can assign default values to parameters:
function greet(name: string = “Guest”): string { return `Hello, ${name}!`; } console.log(greet()); // Output: Hello, Guest! console.log(greet(“Alice”)); // Output: Hello, Alice! |
In this first part of our journey into TypeScript, we’ve explored what makes TypeScript a powerful tool for modern web development. From understanding its core features and setting it up in your project to diving into types and functions, TypeScript brings structure and type safety to JavaScript, enabling developers to write more reliable and maintainable code. Stay tuned for the next part, where we’ll delve deeper into advanced features like interfaces, generics, and more!