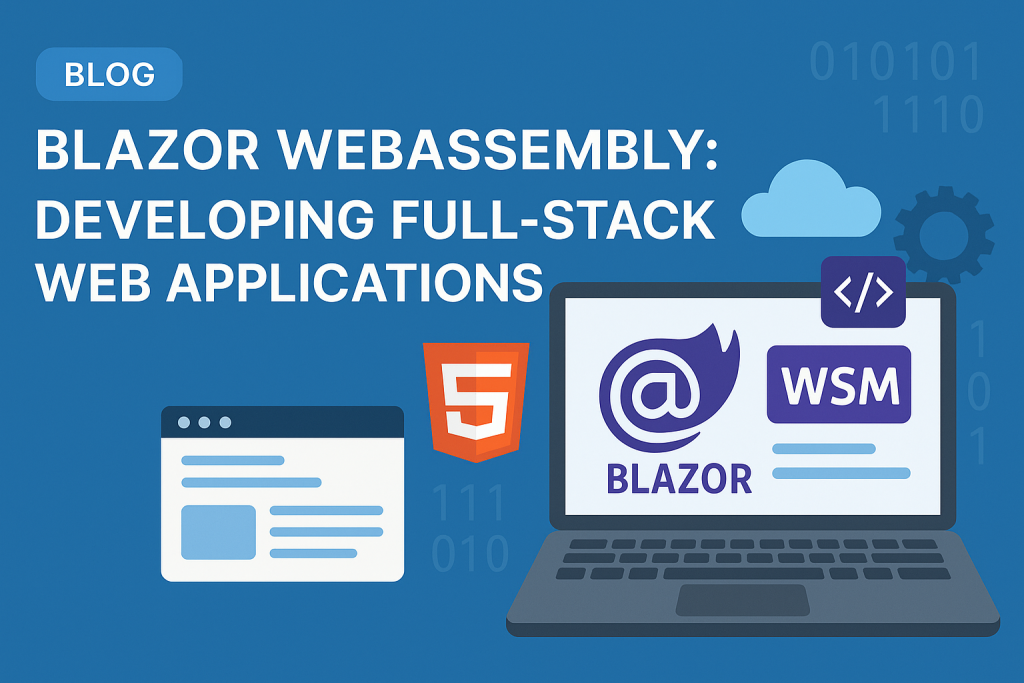
In the ever-evolving landscape of web development, Blazor WebAssembly has emerged as a game-changer. With the power to run C# directly in the browser via WebAssembly, Blazor WebAssembly allows .NET developers to build full stack web applications using a single language — C#. This opens the door for rapid development, improved maintainability, and exceptional performance, all without relying on JavaScript-heavy frontends.
In this in-depth guide, we’ll explore what Blazor WebAssembly is, how it works, and how you can leverage it to build modern, scalable, and high-performance full stack web applications.
What is Blazor WebAssembly?
Blazor WebAssembly (Blazor WASM) is a client-side framework in the ASP.NET Core ecosystem that allows developers to create rich interactive UIs using C# instead of JavaScript. It compiles C# code into WebAssembly — a lightweight binary format that runs at near-native speed in the browser.
Key Features:
- Runs on WebAssembly in the browser with no server dependency for UI interaction.
- C# on both client and server – share models, validation, and business logic.
- Component-based UI development, similar to React or Angular.
- Tight integration with .NET backend, such as ASP.NET Core Web API.
Why Choose Blazor WebAssembly for Full Stack Development?
Blazor WebAssembly allows you to create end-to-end web applications entirely in C# — a major advantage for .NET developers. Here’s why it’s a powerful choice for full stack development:
✅ Single Language Full Stack Development
Blazor allows C# to be used both on the frontend and backend. No need to switch between JavaScript (frontend) and C# (backend). This leads to:
- Reduced context switching
- Fewer bugs
- Shared libraries (DTOs, models, etc.)
✅ Modern Frontend Without JavaScript
You can create highly interactive UIs using Blazor components, event handling, and data binding without writing a single line of JavaScript.
✅ Integration with ASP.NET Core Web API
Blazor WebAssembly can consume RESTful APIs built with ASP.NET Core to enable full stack capabilities — authentication, data operations, real-time communication, etc.
✅ Full Control Over Deployment
Host your app as a static site (e.g., on GitHub Pages, Azure Static Web Apps) or with a .NET backend server (Blazor Server + API combo).
Architecture of a Blazor WebAssembly Full Stack App
[Client (Blazor WASM)] ↔ [Backend (ASP.NET Core API)] ↔ [Database (SQL Server/PostgreSQL)]
Client-Side (Blazor WebAssembly)
- Built with Razor Components (.razor files)
- Handles UI rendering and user interactions
- Calls Web APIs using HttpClient
Server-Side (ASP.NET Core)
- Provides APIs and services to the Blazor client
- Handles data access (via Entity Framework Core or Dapper)
- Implements authentication and business logic
Database Layer
- SQL Server, PostgreSQL, MySQL, or NoSQL (MongoDB, Cosmos DB)
- Accessed via ORM tools like Entity Framework Core
How to Build a Full Stack Web App with Blazor WebAssembly
Let’s walk through the steps to create a basic full stack Blazor WebAssembly app.
1. Create the Solution Structure
dotnet new blazorwasm -o BlazorClient –hosted
cd BlazorClient
This command sets up:
- BlazorClient.Client → Blazor WebAssembly frontend
- BlazorClient.Server → ASP.NET Core backend
- BlazorClient.Shared → Shared models and DTOs
2. Define Shared Models
In BlazorClient.Shared/WeatherForecast.cs:
public class WeatherForecast {
public DateTime Date { get; set; }
public int TemperatureC { get; set; }
public string Summary { get; set; }
}
3. Create API Controller
In BlazorClient.Server/Controllers/WeatherForecastController.cs:
[ApiController]
[Route("api/[controller]")]
public class WeatherForecastController : ControllerBase {
[HttpGet]
public IEnumerable<WeatherForecast> Get() {
return Enumerable.Range(1, 5).Select(index => new WeatherForecast {
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = "Sunny"
});
}
}
4. Consume API in Blazor Client
In BlazorClient.Client/Pages/FetchData.razor:
@inject HttpClient Http
@if (forecasts == null)
{
<p><em>Loading...</em></p>
}
else
{
<table class="table">
@foreach (var forecast in forecasts)
{
<tr>
<td>@forecast.Date.ToShortDateString()</td>
<td>@forecast.TemperatureC</td>
<td>@forecast.Summary</td>
</tr>
}
</table>
}
@code {
private WeatherForecast[]? forecasts;
protected override async Task OnInitializedAsync() {
forecasts = await Http.GetFromJsonAsync<WeatherForecast[]>("api/WeatherForecast");
}
}
Authentication & Authorization in Blazor WebAssembly
Blazor supports robust authentication and authorization mechanisms, including:
- JWT Tokens
- ASP.NET Core Identity
- OAuth / OpenID Connect (OIDC) with Azure AD, Google, or Auth0
With the Microsoft Authentication Library (MSAL), you can implement secure single sign-on (SSO) in minutes.
Performance Optimization Tips
- Enable Ahead-of-Time (AOT) Compilation
- Use lazy loading for assemblies
- Minimize use of JS Interop
- Compress static assets (Brotli, Gzip)
- Use Blazor WebAssembly prerendering with ASP.NET Core
Blazor WebAssembly vs Blazor Server
Feature | Blazor WebAssembly | Blazor Server |
Runs in browser? | ✅ Yes (via WebAssembly) | ❌ No (SignalR connection) |
Offline Support | ✅ Yes | ❌ No |
Latency | ✅ Low (local processing) | ❌ Higher (round-trip to server) |
App Size | ❌ Larger download | ✅ Small initial load |
Complexity | ✅ Easier scaling | ❌ Requires SignalR management |
Popular Use Cases
- Enterprise Dashboards
- Progressive Web Apps (PWAs)
- Admin Panels
- Customer Portals
- IoT & Edge UIs
- Internal Business Apps
Conclusion: Blazor WebAssembly is the Future of Full Stack .NET Development
Blazor WebAssembly empowers .NET developers to build truly modern full stack web applications with high performance, full control, and unmatched productivity — all without leaving the comfort of C#. Whether you’re building internal enterprise tools or rich client-side web apps, Blazor WebAssembly offers the flexibility and power of JavaScript frameworks like React or Angular — without JavaScript.
Ready to Build with Blazor?
Start your journey by exploring Blazor documentation on Microsoft and experimenting with real-world projects. The future of full stack .NET development is already here.