What Are Platform Channels in Flutter?
Platform Channels are a mechanism in Flutter that allows communication between the Dart code (used in Flutter) and the native code of the platform (Android or iOS). This enables Flutter applications to access platform-specific functionality not available in Flutter’s standard library.
Key Features of Platform Channels:
- Two-Way Communication: Allows Flutter and native code to exchange data in both directions.
- Message-Based: Messages are sent using a codec (e.g., JSON, binary) for serialization.
- Customizable: You can define your own method names and data structures.
- Lightweight: Only sends requests for functionality explicitly required, ensuring optimal performance.
Why Use Platform Channels?
Flutter already has many plugins that provide common functionality, but sometimes you may need:
- Access to Device-Specific Features:
For example, accessing hardware like sensors, Bluetooth, or file systems. - Custom Functionality:
Implementing features specific to your app that aren’t available through existing plugins. - Performance Optimization:
Using native code to handle complex or resource-intensive tasks. - Extending Existing Plugins:
Modifying or extending plugin behavior for advanced use cases.
How Do Platform Channels Work?
1. Dart Side (Flutter): Defines a Method Channel and calls methods to send messages to the native platform.
2. Native Side (Android/iOS): Handles these method calls, performs the required operation (e.g., accessing the battery level, invoking native APIs), and sends a response back to Flutter.
Communication Flow:
- Flutter Code:
- Sends a message to the native platform via MethodChannel.
- Native Code
- Receives the message, processes it, and sends a response.
- Flutter Code:
- Receives the response and processes it further in the app.
Detailed Steps with Example: Accessing Battery Level
Use Case:
Display the battery level in your Flutter app using Platform Channels.
1. Flutter Code (Defining the Channel)
Create a MethodChannel in Flutter to interact with the native platform.
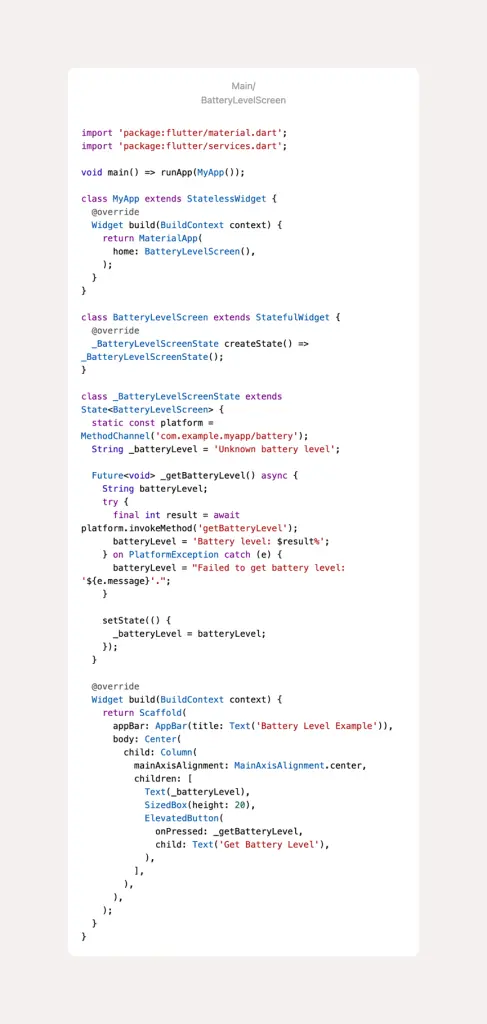
2. Android Code (Kotlin)
Steps:
- Open android/app/src/main/kotlin/<your_package_name>/MainActivity.kt.
- Configure the MethodChannel to listen for the getBatteryLevel method.
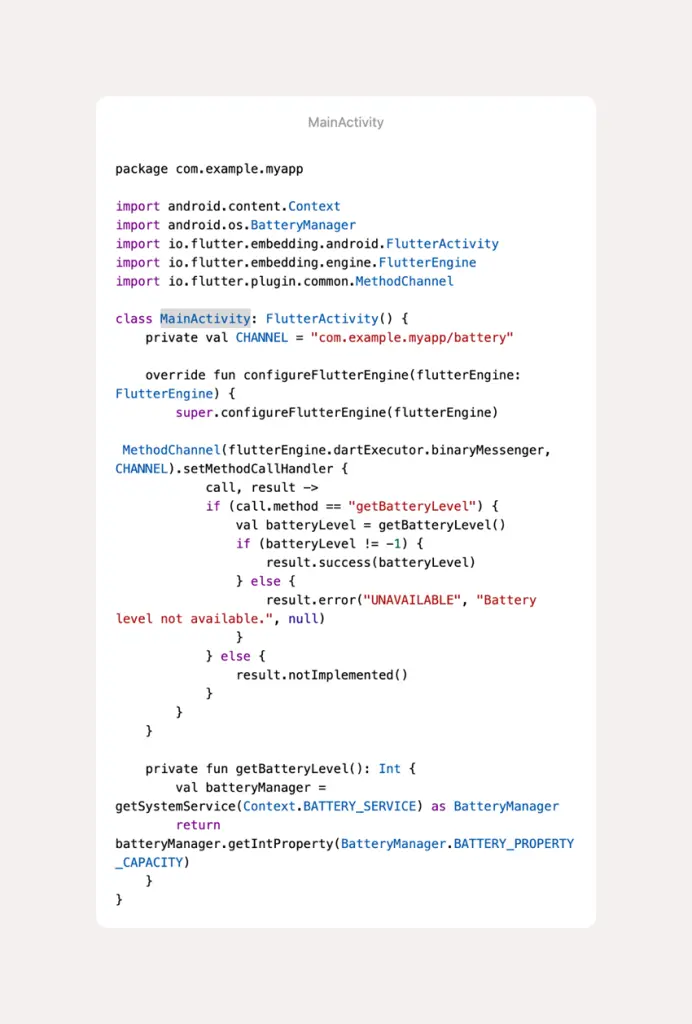
3. iOS Code (Swift)
Steps:
- Open ios/Runner/AppDelegate.swift.
- Configure the FlutterMethodChannel to handle the getBatteryLevel method.
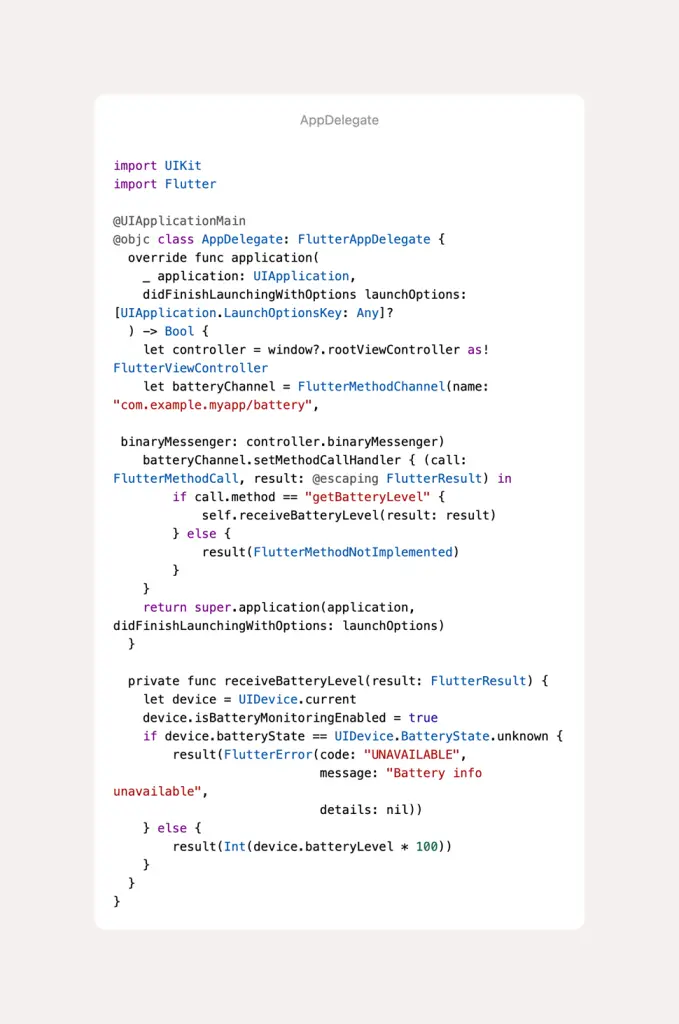
4. Testing the Integration
- Run the app on an emulator or real device.
- Tap the “Get Battery Level” button to fetch the battery percentage.
Output
- When the button is clicked, the app displays the battery percentage:
- “Battery level: 76%”
Best Practices
- Error Handling:
- Handle PlatformException in Flutter and provide meaningful messages.
- Use Unique Channel Names:
- Ensure your channel names are unique to avoid conflicts in larger projects.
- Keep Native Code Clean:
- Isolate the native logic into separate utility functions or classes to enhance readability.
- Test on Real Devices:
- Emulators may not accurately reflect hardware-specific behaviors like battery level.
Here are a few helpful references for understanding and implementing Flutter Platform Channels:
- DhiWise: This guide explains how to set up a MethodChannel in Dart and communicate with native Android (Kotlin) and iOS (Swift) code. It provides examples such as fetching the device’s battery level. A detailed step-by-step process with code snippets is included.
- Read more at DhiWise DhiWise.
- Flutter Official Docs: The official documentation by Flutter explains how to use platform channels to access native features. It covers the use of MethodChannel for synchronous communication and provides guidance on binary message codecs for advanced use cases. View Flutter’s official documentation DECODE.
These resources cover foundational concepts, practical examples, and advanced use cases, making them excellent starting points for mastering Flutter Platform Channels.
Conclusion
- Summarize the importance of Platform Channels in bridging the gap between Flutter and native code.
- Encourage readers to explore Platform Channels for advanced app features.