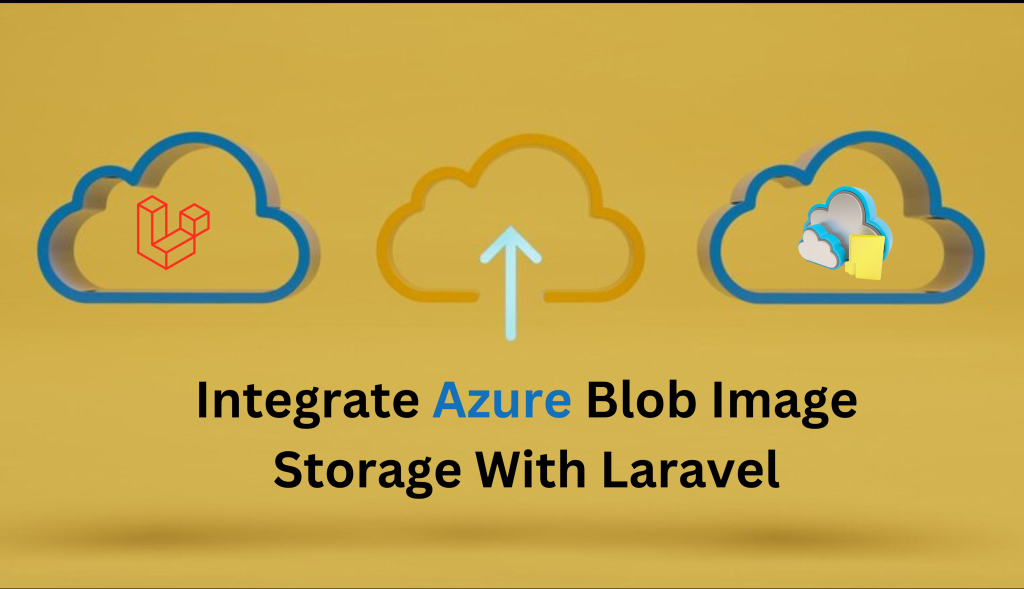
Azure Blob Storage is a cloud-based service from Microsoft Azure designed for storing massive amounts of untrusted data, such as images, videos, documents, and backups.
It is ideal for web applications that need scalable, secure, and globally accessible storage.
When integrated with Laravel, Azure Blob Storage becomes an excellent solution for managing media files and other assets. Laravel’s powerful filesystem abstraction, combined with the Fly system adapter for Azure, allows developers to seamlessly interact with Azure Blob Storage as if it were a local or S3- compatible disk.
Step 1: Install Required Packages
Install the microsoft/azure-storage-blob package to interact with Azure Blob Storage:
- composer require microsoft/azure-storage-blob
Install the league/flysystem-azure-blob package for Flysystem compatibility:
- composer require league/flysystem-azure-blob
Step 2: Configure Azure Blob Storage in Laravel
- Add your Azure Blob Storage credentials to the .env file:
-AZURE_STORAGE_ACCOUNT_NAME=
-AZURE_STORAGE_ACCOUNT_KEY=<your-account-key>
-AZURE_STORAGE_CONTAINER=<your-container-name>
-AZURE_STORAGE_URL=https://<your-storage-account- name>.blob.core.windows.net/
- Update the config/filesystems.php file to include the Azure Blob Storage configuration:
'disks' => [
// Other disk configurations...
'azure' => [
'driver' => 'azure',
'name' => env('AZURE_STORAGE_ACCOUNT_NAME'),
'key' => env('AZURE_STORAGE_ACCOUNT_KEY'),
'container' => env('AZURE_STORAGE_CONTAINER'),
'endpoint' => env('AZURE_STORAGE_URL'),
],
],
Step 3: Use Azure Blob Storage in Laravel
- Uploading Files to Azure Blob Storage: Use Laravel’s Storage facade to upload files to the Azure Blob Storage container:
use Illuminate\Support\Facades\Storage;
$filePath = $request->file('image')->store('images', 'azure'); return response()->json(['path' => $filePath], 201);
- Retrieving Files from Azure Blob Storage: Generate a URL for a stored file:
$fileUrl = Storage::disk('azure')->url('images/example.jpg');
return response()->json(['url' => $fileUrl]);
- Deleting Files: Delete a file from the Azure Blob Storage container:
Storage::disk('azure')->delete('images/example.jpg');
- Listing Files in a Container: Retrieve all files in a directory:
$files = Storage::disk('azure')->files('images');
return response()->json(['files' => $files]);
Advantages of Using Azure Blob Storage in Laravel
- Scalability: Handle large amounts of data with ease, perfect for applications dealing with high volumes of images or files.
- Global Accessibility: Azure Blob Storage ensures data availability worldwide with replication options.
- Security: Protect your data with Azure’s advanced encryption and access control mechanisms, including SAS tokens and private containers.
- Cost Optimization: Store files in different access tiers (Hot, Cool, Archive) to minimize costs based on data usage patterns.
- Seamless Integration: Laravel’s Storage facade works effortlessly with Azure Blob Storage, making file management intuitive and developer-friendly
Why Choose Laravel for Azure Blob Storage?
Laravel’s built-in tools and abstractions simplify working with external storage services. With Azure Blob Storage, Laravel developers can:
- Upload files dynamically using the Storage facade.
- Retrieve and generate URLs for public files.
- Delete files directly from the Blob container.
- Manage complex storage operations like batch uploads or directory handling
Conclusion: –
With Laravel’s robust file system abstraction and Azure’s scalable Blob Storage, you can easily handle image uploads, retrievals, and management.
The combination ensures your application is ready for large-scale image storage requirements.