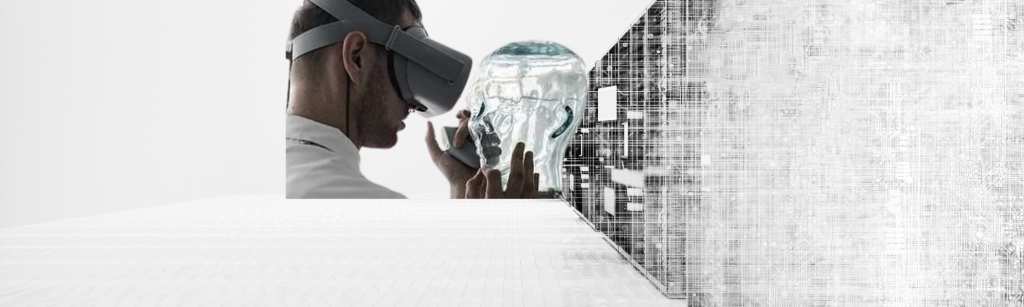
Introduction
Apple’s vision for the future of computing is evolving rapidly with the introduction of visionOS and the groundbreaking Apple Vision Pro. These innovations are reshaping the way we interact with digital content by merging the physical and digital worlds. In this blog, we explore how visionOS extends the iOS ecosystem into immersive spatial experiences, discuss how developers can build captivating augmented, mixed, and virtual reality apps, and provide practical coding examples using Swift, SwiftUI, and RealityKit. High-volume keywords such as visionOS, Apple Vision Pro, immersive experiences, spatial computing, AR/VR development, and future of iOS are woven throughout this guide to ensure you capture all the insights you need to be at the forefront of this new era.
What is visionOS?
visionOS is Apple’s new operating system designed specifically for spatial computing devices like the Apple Vision Pro. Built to create immersive, 3D experiences that blend digital content with the real world, visionOS is a natural evolution of iOS—leveraging familiar tools while opening up entirely new ways to interact with apps. With visionOS, developers can build apps that support intuitive gestures, spatial audio, and dynamic interactions that make the digital world feel tangible.
Key Highlights of visionOS:
- Immersive 3D Environments: Create experiences that place digital objects in real space.
- Natural User Interfaces: Utilize hand tracking, eye tracking, and voice commands.
- Unified Ecosystem: Seamlessly integrate with existing iOS apps and services.
- Spatial Interactions: Allow users to interact with content in a natural, intuitive manner.
visionOS is not meant to replace iOS but to expand its horizons—bringing the beloved iOS development ecosystem into the era of spatial computing.
The Future of iOS in a Spatial World
With the advent of visionOS and Apple Vision Pro, the iOS landscape is set to undergo a paradigm shift. The future of iOS is not just about smaller screens and touch gestures anymore—it’s about integrating 2D interfaces with 3D immersive experiences.
What Does This Mean for Developers?
- Cross-Platform Synergy: Leverage your existing iOS skills and codebase while exploring new spatial UI paradigms.
- Extended Reality (XR) Applications: Build augmented reality (AR), virtual reality (VR), and mixed reality (MR) apps that provide enriched user experiences.
- Innovative UI/UX Design: Experiment with depth, perspective, and interactive 3D elements to captivate users.
- Unified Development Environment: Use tools like Swift, SwiftUI, RealityKit, and ARKit to create consistent, innovative apps across iOS and visionOS.
The move towards spatial computing represents a convergence of digital and physical worlds, setting the stage for apps that are more interactive, intuitive, and engaging than ever before.
Building Immersive Experiences for Apple Vision Pro
Developing for visionOS and Apple Vision Pro means embracing new design paradigms. Here’s how you can get started:
1. Embrace SwiftUI and RealityKit
Apple’s SwiftUI continues to be a powerful declarative framework for building user interfaces. When combined with RealityKit, you can easily create, manipulate, and render 3D content.
Example: A Basic SwiftUI + RealityKit Scene
Below is a simple Swift code snippet that creates a basic immersive scene using RealityKit. This example sets up an ARView (which will soon evolve to support visionOS) and places a 3D box in the scene.
import SwiftUI
import RealityKit
import ARKit
struct ImmersiveView: UIViewRepresentable {
func makeUIView(context: Context) -> ARView {
let arView = ARView(frame: .zero)
// Configure AR session for world tracking (adaptable for visionOS)
let configuration = ARWorldTrackingConfiguration()
arView.session.run(configuration)
// Create a simple box entity
let boxMesh = MeshResource.generateBox(size: 0.2)
let material = SimpleMaterial(color: .blue, isMetallic: true)
let boxEntity = ModelEntity(mesh: boxMesh, materials: [material])
// Set the box's position
boxEntity.position = SIMD3(0, 0, -0.5)
// Create an anchor and add the box entity
let anchorEntity = AnchorEntity(world: .zero)
anchorEntity.addChild(boxEntity)
arView.scene.addAnchor(anchorEntity)
return arView
}
func updateUIView(_ uiView: ARView, context: Context) {}
}
struct ContentView: View {
var body: some View {
ImmersiveView()
.edgesIgnoringSafeArea(.all)
}
}
@main
struct VisionOSDemoApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
This code demonstrates how to create a 3D scene using RealityKit integrated with SwiftUI. While this example is written with ARKit in mind, the transition to visionOS will allow similar code to power spatial apps for Apple Vision Pro with additional spatial interaction capabilities.
2. Designing for Spatial Interactions
When building immersive experiences, consider how users will interact with your app:
- Gestural Input: Use hand and finger gestures to interact with virtual objects.
- Eye Tracking and Focus: Create dynamic interfaces that respond to where users are looking.
- Voice Commands: Integrate Siri and natural language processing to allow voice-based control.
- Depth and Perspective: Design interfaces that take advantage of 3D space—elements can float, scale, or rotate based on user context.
3. Integrating ARKit Enhancements
For developers transitioning from traditional AR experiences to visionOS, ARKit continues to evolve. Enhanced tracking, object detection, and environmental understanding help create more realistic and context-aware experiences.
Example: Reacting to User Gestures
Imagine an app where a user can tap on a virtual object to trigger an animation. Using Swift’s Combine framework along with gesture recognizers, you can build responsive experiences. Here’s a conceptual snippet:
// Pseudo-code for handling tap gestures on a 3D entity in RealityKit
let tapGesture = UITapGestureRecognizer(target: context.coordinator, action: #selector(context.coordinator.handleTap(_:)))
arView.addGestureRecognizer(tapGesture)
// In your coordinator:
@objc func handleTap(_ sender: UITapGestureRecognizer) {
let location = sender.location(in: arView)
if let entity = arView.entity(at: location) {
// Perform an animation or interaction on the entity
entity.move(to: Transform(translation: SIMD3(0, 0.2, 0)), relativeTo: entity, duration: 0.5)
}
}
This example outlines how you might detect a tap and trigger an animation, a pattern that can be adapted to spatial interactions on visionOS.
Best Practices for Developing Immersive Experiences
1. Optimize for Performance
- Efficient Rendering: Optimize 3D assets to reduce draw calls and maintain high frame rates.
- Resource Management: Use lazy loading for heavy assets and optimize memory usage.
- Profiling Tools: Leverage Xcode’s performance tools to monitor CPU/GPU usage and optimize your code.
2. Prioritize User Comfort
- Motion Sickness Prevention: Design smooth transitions and avoid rapid movements or unexpected changes in perspective.
- Ergonomic Design: Consider the physical comfort of prolonged use. Interfaces should minimize eye strain and allow for natural interactions.
- Accessibility: Incorporate voice, haptic feedback, and scalable UI elements to ensure inclusivity.
3. Test Extensively
- Simulators and Real Devices: Use Apple’s simulators for visionOS and, when available, test on actual hardware (Apple Vision Pro) to fine-tune the user experience.
- User Feedback: Gather feedback early through beta testing and iterate based on user insights.
The Future of visionOS and iOS
As Apple continues to push the boundaries of spatial computing with visionOS, the future of iOS development is set to become more immersive, interactive, and integrated with our physical environments. Here are some trends to watch:
- Convergence of 2D and 3D: Expect a seamless blend of traditional iOS apps with spatial interfaces.
- Enhanced Developer Tools: More advanced APIs and frameworks will simplify the creation of immersive apps.
- Broader Adoption of Spatial Computing: As devices like Apple Vision Pro become mainstream, immersive experiences will become a key differentiator in app development.
- Innovative Interaction Paradigms: With support for hand tracking, eye tracking, and voice commands, new interaction models will redefine user engagement.
Developers who adapt early and embrace these innovations will be well-positioned to create the next generation of groundbreaking apps that capture the imagination and transform the way we interact with technology.
Conclusion
visionOS and the Apple Vision Pro mark the beginning of a new era for iOS and spatial computing. By harnessing the power of immersive technologies, developers can create experiences that are not only visually stunning but also deeply intuitive and user-centric. With tools like SwiftUI, RealityKit, and ARKit—along with emerging frameworks designed specifically for visionOS—the future of mobile app development is here.
Embrace this exciting opportunity to extend your iOS expertise into the realm of spatial computing. Whether you’re building for augmented reality, virtual reality, or mixed reality, the principles remain the same: deliver seamless, engaging, and performant experiences. By following best practices, leveraging high-performance coding techniques, and keeping user comfort in mind, you’ll be at the forefront of the immersive computing revolution.
The journey into spatial computing is just beginning. As Apple continues to innovate and the ecosystem evolves, stay agile, keep experimenting, and be ready to reimagine what apps can do in three-dimensional space. The future of iOS is immersive, and the possibilities are limitless.
Happy coding, and welcome to the next frontier of immersive app development!